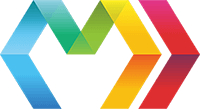
The @marko/webpack/loader loader for Webpack will automatically compile all imported Marko templates during bundling. In addition, it will automatically bundle any template dependencies (including required CSS).
ProTip: Want to see it in action? Check out the
marko-webpack
demo repository. Or runnpx @marko/create --template webpack
to use this sample as a starting point for a new app.
npm install markonpm install webpack @marko/webpack --save-dev
Let's say we have a simple view that we want to render in the browser: hello.marko
<h1>Hello </h1>
h1 -- Hello
First, let's create a client.js
that requires the view and renders it to the body:
;MyTemplate;
Now, let's configure webpack
to compile the client.js
file and use @marko/webpack/loader
for any *.marko
files:
moduleexports =entry: "./client.js"output:path: __dirnamefilename: "static/bundle.js"resolve:extensions: ".js" ".marko"module:rules:test: /\.marko$/loader: "@marko/webpack/loader";
Run webpack
from your terminal and you'll have a new static/bundle.js
file created. Reference that from an html file and you're good to go.
Load up that page in your browser and you should see Hello Marko
staring back at you.
If you're using inline css with pre-processors, you must configure the appropriate loader.
style.less {.prettycolor:@pretty-color;<div.pretty/>
style.less {.prettycolor: @pretty-color;div.pretty
//...module:rules://...test: /\.less$/ // matches style.less { ... } from our templateuse: "style-loader" "css-loader" "less-loader"//...;//...
It is recommended to configure the MiniCSSExtractPlugin
so that you get a separate css bundle rather than it being included in the JavaScript bundle.
npm install mini-css-extract-plugin --save-dev
EDITconst CSSExtractPlugin = ;moduleexports =entry: "./client.js"resolve:extensions: ".js" ".marko"module:rules:test: /\.marko$/loader: "@marko/webpack/loader"test: /\.$/use: CSSExtractPluginloader "css-loader" "less-loader"plugins:// Write out CSS bundle to its own file:filename: "[name].css";
Helpful? You can thank these awesome people! You can also edit this doc if you see any issues or want to improve it.