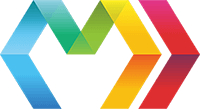
To render a Marko view, you need to require
it.
var fancyButton = ;
Note: If you are targeting node.js, you will need to enable the require extension in order to require
.marko
files or you will need to precompile all of your templates using Marko CLI. If you are targeting the browser, you will need to use a bundler likelasso
,webpack
,browserify
orrollup
.
Once you have a view, you can pass input data and render it:
var button = ;var html = button;console;
The input data becomes available as input
within a view, so if fancy-button.marko
looked like this:
<button></button>
button --
The output HTML would be:
Click me!
We used the renderToString
method above to render the view, but there are a number of different method signatures that can be used to render.
Many of these methods return a RenderResult
which is an object with helper methods for working with the rendered output.
renderSync(input)
params | type | description |
---|---|---|
input | Object | the input data used to render the view |
return value | RenderResult | The result of the render |
Using renderSync
forces the render to complete synchronously. If a tag attempts to run asynchronously, an error will be thrown.
var view = ; // Import `./view.marko`var result = view;result;
render(input)
params | type | description |
---|---|---|
input | Object | the input data used to render the view |
return value | AsyncStream /AsyncVDOMBuilder | the async out render target |
The render
method returns an async out
which is used to generate HTML on the server or a virtual DOM in the browser. In either case, the async out
has a then
method that follows the Promises/A+ spec, so it can be used as if it were a Promise. This promise resolves to a RenderResult
.
var view = ; // Import `./view.marko`var resultPromise = view;resultPromise;
render(input, callback)
params | type | description |
---|---|---|
input | Object | the input data used to render the view |
callback | Function | a function to call when the render is complete |
callback value | RenderResult | The result of the render |
return value | AsyncStream /AsyncVDOMBuilder | the async out render target |
var view = ; // Import `./view.marko`view;
render(input, stream)
params | type | description |
---|---|---|
input | Object | the input data used to render the view |
stream | WritableStream | a writeable stream |
return value | AsyncStream /AsyncVDOMBuilder | the async out render target |
The HTML output is written to the passed stream
.
var http = ;var view = ; // Import `./view.marko`http;
render(input, out)
params | type | description |
---|---|---|
input | Object | the input data used to render the view |
out | AsyncStream /AsyncVDOMBuilder | The async out to render to |
return value | AsyncStream /AsyncVDOMBuilder | The out that was passed |
The render
method also allows passing an existing async out
. If you do this, render
will not automatically end the async out
(this allows rendering a view in the middle of another view). If the async out
won't be ended by other means, you are responsible for ending it.
var view = ; // Import `./view.marko`var out = view;view;out;out;
renderToString(input)
params | type | description |
---|---|---|
input | Object | the input data used to render the view |
return value | String | The HTML string produced by the render |
Returns an HTML string and forces the render to complete synchronously. If a tag attempts to run asynchronously, an error will be thrown.
var view = ; // Import `./view.marko`var html = view;documentbodyinnerHTML = html;
renderToString(input, callback)
params | type | description |
---|---|---|
input | Object | the input data used to render the view |
callback value | String | The HTML string produced by the render |
return value | undefined | N/A |
An HTML string is passed to the callback.
var view = ; // Import `./view.marko`view;
stream(input)
The stream
method returns a node.js style stream of the output HTML. This method is available on the server, but is not available by default in the browser. If you need to use streams in the browser, you may require('marko/stream')
as part of your client-side bundle.
var fs = ;var view = ; // Import `./view.marko`var writeStream = fs;view;
getComponent()
getComponents(selector)
afterInsert(doc)
getNode(doc)
getOutput()
appendTo(targetEl)
insertAfter(targetEl)
insertBefore(targetEl)
prependTo(targetEl)
replace(targetEl)
replaceChildrenOf(targetEl)
If you need to make data available globally to all views that are rendered as the result of a call to one of the above render methods, you can pass the data as a $global
property on the input data object. This object will be removed from input
and merged into the out.global
property.
view;
To prevent sensitive data to be accidentally shipped to the browser, by default none of the keys in out.global
is going to be sent to the browser. If you want the data to be serialized and ship to the frontend you need to specify it in serializedGlobals
inside the $global
object and they persist across re-renderings. The values need to be serializable.
app;
Use $global
with judgement. It is global and visible in any component.
Check this PR for more details.
EDITHelpful? You can thank these awesome people! You can also edit this doc if you see any issues or want to improve it.