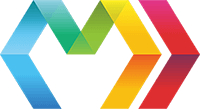
See the marko-redux
sample project for a fully-working example.
First, save the marko
and redux
packages to your project’s dependencies:
npm i marko redux
The partial code below shows how a Marko UI component can connect to a Redux store, using Redux’s store.subscribe()
method and Marko’s forceUpdate()
method:
counter.marko
importclass<counterstore.getState />
importclasscounterstore.getState
reducer.js
module {state = state || value: 0 ;// Additional reducer logic here…return state;};
store.js
In counter.marko
, the imported store module exports a Redux store created with the following code:
EDITconst redux = ;const counter = ;moduleexports = redux;
Helpful? You can thank these awesome people! You can also edit this doc if you see any issues or want to improve it.